Syntax
php tag
<?php [our code] >
variables
$variable_name = value;
Object oriented PHP
objects
unique instance of a class
creating a new object
new
ex. $app = new \Slim\Slim();
ex. $physics = new Books;
class
Basic
implements
a template that includes datatypes, functions etc,
objects are based on classes
<?php class name{ var $varName = 'value'; }; ?>
member variable
variable created inside class
by default it is accesible outiste the class
accesed by member function
$viariable->
variable + object operator
$log->addWarning('log');
member function
function created inside class
in OOP function is called a method
calling mf
you can call it after creating new object
$physics->setTitle("Physics for High School");
Subtopic
$physics->setPrice(15);
$physics->getTitle(); $physics->getPrice();
constructor
special type of function
is called automaticly when new object is created
is usefull to construct new object
ex.
remember! when writing inside constructor $this
$this->name = $name;
using parent constructor
we can use constructor form parent function to make our code DRY
ex.
descructor
__destruct()
limiting accesibility to class properties and metods
private
it limits accesibility to the class in with it is declared
ex.
inherited class can'not acces private stuff
protected
protected stuff is accessible only form parent, and child class
ex.
final keywords
prevent parent methods and properties from beeing override by child class
ex.
giving access to calss properties and methods
static keywords
make methods and properties accessible without creating new instance of class
setting
accessing
public
it is set by default
gives acces to your mrthods and properties form outsite the class
abstract classes
those classes can only be inherited. Exists only virtually
can contain abstract methods
only abstract class can contain abstract methods
ex.
constansts
like a variable but once you declare it, it never changes value
without $
ex.
calling it
interfaces
way of easy addnig interface functions to new implementations of classes
first we craete interface
then we implements it to class
inheritance
for creating new-child class based on parent class
child class will inherit all variables, and functions from parent class
and also extends parent class with new variables nad functions
ex.
function overriding
function inherited form parent class can be modified
ex.
tools & tricks
method_exist(object, method_name)
it can check if method exist inside a class
ex.
ex2.
is_subclass_of(object, class_name)
check if object is the child of a parent class
like wih DNA samples :)
ex.
make it short with variables
ex.
class_exist()
is_a()
MVC
View
Front-End
what the user see
HTML+CSS+Forms
Cotroller
controls the flow of information between...
Model
COntain database related code
Composer - dependency menager
composer.json
autoload
allows us to load automaticly requided classes, when we use the name of class in our code :)
can by made simplu with composer
Slim Microframework
instaling via composer
composer require
slim
0
basic instance of routing
it takes argument form URL, and use it in the function or anything
routing based on URL
but
we need to create .htaccess file to tell apache to route all traffic to our site through the index.php file
to point at html file
post data validation
from simple contact form
ex.
if there is an error
$app->redirect('/contact');
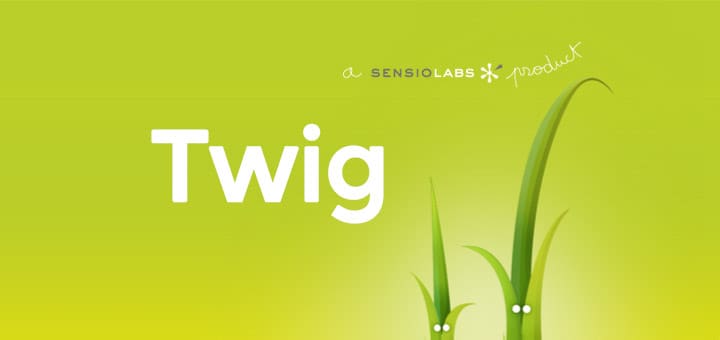
Twig
PHP templeting language
insall with composer
composer require
twig
0
including it to slim/views
composer require
in our index.php
setting options for twig
debbuging
setting twig extension
helpers like urlFOR, siteURL, baseURL, currentURL
baseURL
link for a main domain
{{ baseUrl() }}
siteURL
<a href="{{ baseUrl() }}" >Home</a>
<a href="{{ siteUrl('/contact') }}">Contact</a>
ex, making multiple list items in navigation
setting vairiable in html
{% set name = 'Hampton' %}
echoing sth
{{ it appers on the screen }}
run some code
{% code %}
variables goeas without $
filters
uppercase
functions
for function - range
control structure - if
including templates
{% include 'sidebar.html' %}
comments
{# comments goes here #}
DRY our HTML
every site with unique content
{% extends 'main.twig' %}
then {% block content %}
functions
echo 'Hello World';
like console.log in JS
require 'folder/package_name';
including sth into our project
date
date_default_timezone_set($timezone_identifier);
sets time for ex. in case of log to my timezone
use Path\Name\File;
setting path like a variable on the begining save us from repeting it in our code (only the file name in our code)
var_dump()
gives us information about variable
ex.
empty()
checks if sth is empty or not
!empty($varName)
ex.
filter_var($varName, filter)
filters variable with a specified filter
validate filters
sanitize filters
they remove all the characters we don't want
FILTER_SANITIZE_STRING
FILTER_SANITIZE_EMAIL
others
ex.
PSR
Basic coding standards
special
variables
$this
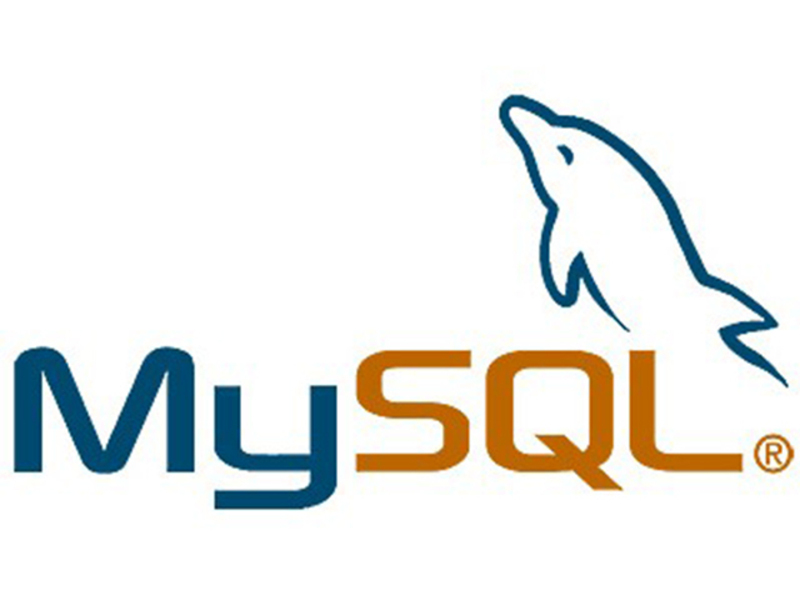
DATATYPES
Strings
TEXT
VARCHAR
CHAR
Numbers
FLOAT
DECIMALS
INTEGERS
Data
TIME
DATA
DATATIME
YEAR
TIMESTAMP
Format: YYYY-MM-DD HH:MI:SS
with seconds
NULL
no data
= oparator not working
you have to use IS
ex. WHERE year IS NULL;
filtering null
WHERE year IS NOT NULL
KEYS
Primary keys
id
integers
ex. CREATE TABLE genres (id INTEGER [AUTO_INCREMENT]PRIMARY KEY, name VARCHAR(50));
Unique keys
email_adress
ssn
set of numbers ex. seciuruty number
CREATE TABLE genres (id INTEGER [AUTO_INCREMENT]PRIMARY KEY, name VARCHAR(50) UNIQUE);
Foreign keys
aka. reference keys
relationschip between two tables
ex. ALTER TABLE movies
ADD COLUMN genre_id INTEGER NULL,
ADD CONSTRAINT FOREIGN KEY (genre_id) REFERENCES genre(id);
Can't be null or duplicated
QUERIES
DDL
USE movies_db_1;
select the database
CREATE
CREATING DATABASES
CREATE SCHEMA 'movies_db_1';
creates a new database
CREATE DATABASE 'movies_db_1';
is possible
CREATE DATABASE IF NOT EXISTS 'movies_db_1';
if yes it will show an warning, not an error
DEFAULT CHARACTER SET utf8;
for setting uncode
or CHARACTER SET = utf8;
CREATING TABLES
CREATE TABLE movies (title VARCHAR(200), year INTEGER);
CREATE TABLE actors (name VARCHAR (50));
CREATE TEMPORARY TABLE;
accesible only for the connection
column definitions
(name VARCHAR(50) NOT NULL);
it don't let you left the field empty;
NULL
it is the dafault value
defining an engine
ex. ...ENGINE = InnoDB;
SHOW ENGINES;
listeing diffrent things
default engine from MySQL 5 is InnoDB
RENAMING TABLES
RENAME TABLE old_table_name TO new_table_name;
DELETING TABLES
DROP TABLE movie_table;
DROP TABLE IF EXIST table_name;
warrning not an error
CLEANING TABLES
TRUNCATE [TABLE] movies_table;
it deletes all the table nat creates new
ALTERING COLUMNS
ALTER TABLE table_name ADD [COLUMN] column_name VARCHAR(100);
ALTER TABLE actors_table ADD (pob VARCHAR(100), dob DATE);
ALTER TABLE actors_table CHANGE [COLUMN] pob place_of_birth VARCHAR(100);
ALTER TABLE actors_table DROP date_of_birth;
DELETING DATABASE
DROP DATABASE | SCHEMA [IF EXISTS] movies_db;
DML (CRUD)
SELECT
SELECT * FROM movies;
filtering data like from objects
ex. movies.title
order is important!
INSERT
INSERT INTO movies VALUES ("Avatar",2009);
INSERT INTO movies VALUES ('Avatar', 2009), ('Avatar 2'), NULL);
multiple
INSERT INTO movies SET title = "Back to the Future", year = 1985;
INSERT INTO users (username, email, first_name, last_name)
VALUES ("henry", "henry@email.com", "Henry", "Chalkley");
UPDATE
UPDATE movies SET year=2015 WHERE title="Avatar 2";
UPDATE movies SET year=2016, title = "Avatar Reloaded" WHERE title="Avatar 2";
DELETE
DELETE FROM movies WHERE title = "Avatar 2" AND year = 2016;
SYMBOLS
*
all data from
WHERE
adds a condition
ex. WHERE year = 1999;
OPERATORS
=
test for condition
!
not equal
ex. WHERE year != 1999;
< >
LESS, MORE than sth
<= >=
or equal to
AND
...another condition
OR
if first condition is not meet
BETWEEN
range of
ex. WHERE year BETWEEN 1999 AND 2004;
LIKE
part of a string
ex. WHERE title LIKE "gotfather";
%
wildcard
ex. WHERE title LIKE "%gotfather";
ex. WHERE title LIKE "%gotfather%";
ORDER BY
uporządkowane według
ex. ORDER BY year;
DESC
descending
from large to small
ASC
ascending
from small to large
ex. SELECT * FROM movies ORDER BY year ASC, title DESC;
LIMIT
limit the results to given number
LIMIT 20, 10;
offset 20, limit 10
OFFSET
0
counting from 0
1
counting from 1
AUTO_INCREMENT
for integers
increment number by one after creating a new row
Column order
FIRST
add a column as a first column in table
ex. ALTER TABLE movies ADD COLUMN id INTEGER AUTO_INCREMENT PRIMARY KEY FIRST;
AFTER column_name
CONSTRAINT
By this keyword A FOREIGN KEY in one table points to a PRIMARY KEY in another table
Joining columns
INNER JOIN
SELECT * FROM movies INNER JOIN genres ON movies.genre_id = genres.id;
OUTER JOIN
SELECT * FROM movies [LEFT | RIGHT] OUTER JOIN genres ON movies.genre_id = genres.id;
ALIAS
SELECT movies.title, genres.name AS genre_name...
temporary gives the table name we want
Functions
COUNT
SELECT COUNT (*) FROM rewievs WHERE movie_id = 1;
shows us how many reviews the film has
MIN
SELECT MIN(score) AS minimum_score FROM reviews WHERE movie_id = 1;
shows the minimum score for the film
MAX
SELECT MAX(score) AS maximum_score FROM reviews WHERE movie_id = 1;
shows the maximum score for the film
SUM
SELECT SUM(score) WHERE movie_id = 1;
AVG
SELECT AVG(score) AS average WHERE movie_id = 1;
GROUP BY
shows us grupped results by content of chosen column
IFNULL
IFNULL (AVG(score),0)
gives us 0 instead of null
HAVING
way of filtering after GRUPING and AGREGATING
GRUP BY movies_id HAVING average > 3;
SELECT title, MIN(score) AS minimum_score,
MAX(score) AS maximum_score,
AVG(score) AS average
FROM movies LEFT OUTER JOIN reviews
ON movies.id = reviews.movie_id
WHERE year_released > 1999
GROUP BY movie_id HAVING average > 3;
long jonhson...
Filtering string results
LOWER(email)
shows column in lowercase
UPPER(last_names)
LENGHT(username)
shows column with length
CONCAT(first_name, UPPER(last_name)) AS full_name
SUBSTRING(email, 1, 10)
it gives us a partial email to 10 character
SQL is 1 based index
ex. CONCAT(SUBSTRING(LOWER(email), 1,10), "...") AS partial_email
andrew@cha...
," ",
adds a space inbetween
EXPLAIN SELECT * ...
gives us a raport about the query
INDEXING
CREATE INDEX index_name ON users(last_name);
Subtopic
SETTINGS
Turn off the safe updates mode
SET SQL_SAFE_UPDATES = 0;
CREATING USERS
Permission to read
GRANT SELECT ON database_name. * TO user1@'%' IDENTIFIED BY 'password';
'%' is a wildcart to host location of a user
user1 can connect from any IP
Permission to read and write, delete (all CRUD)
GRANT SELECT, INSERT, UPDATE, DELETE ON treehouse_movie_db.*
TO user2@'127.0.0.1'
IDENTIFIED BY 'password';
DDL permissions
GRANT ALTER CREATE DROP ON treehouse_movie_db.*
TO user3@'127.0.0.1'
IDENTIFIED BY 'password';
FLUSH PRIVILEGES;
obligatory! it resets and updates premisions